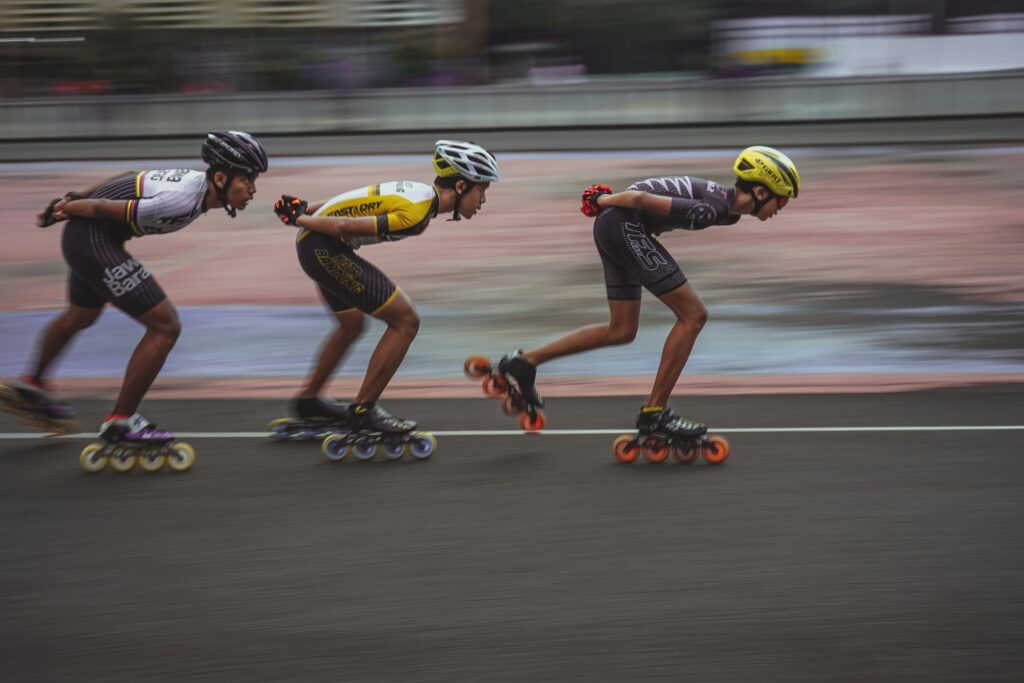
Ansible is a powerful automation tool that makes it easy to manage and configure servers, applications, and infrastructure. As your infrastructure expands, your Ansible playbooks become more complex and time-consuming. Keeping Ansible running efficiently is crucial for maintaining a responsive and scalable environment. Here are some practical suggestions for optimizing Ansible for faster performance.
1. Optimize Inventory Management
Ansible’s inventory management is the starting point for optimizing playbook execution:
- Dynamic Inventory: Use dynamic inventory scripts to generate your inventory on the fly, especially in cloud environments. This reduces the overhead of maintaining static inventory files and ensures up-to-date host information. here a link how can you use terraform and ansible to generate Dynamic Inventory.
- Limit Scope: Use the
--limit
option to restrict playbook execution to specific hosts or groups. This avoids unnecessary tasks on unrelated hosts.
2. Reduce SSH Overhead
Ansible communicates with remote hosts primarily over SSH, so optimizing SSH can lead to significant performance gains:
- Control Persist: Enable SSH connection multiplexing to reuse SSH connections, reducing the time spent establishing connections. Add the following to your SSH configuration (
~/.ssh/config
):
Host *
ControlMaster auto
ControlPath /tmp/ssh-%r@%h:%p
ControlPersist 10m
- Parallelism: Increase the number of parallel SSH connections using the
-f
or--forks
option. The default is 5, but you can increase it based on your infrastructure capabilities:
ansible-playbook -f 10 playbook.yml
3. Efficient Playbook Design
Designing your playbooks efficiently can significantly reduce execution time:
- Task Optimization: Combine related tasks to minimize the number of remote executions. For example, instead of running multiple commands individually, use a shell script or a command with multiple arguments.
- Idempotence: Ensure tasks are idempotent, meaning they only make changes when necessary. This reduces redundant operations and speeds up re-runs. For example, run apt update only if the “Download nginx apt key” task is changed.
- name: Download nginx apt key
get_url:
url: http://nginx.org/keys/nginx_signing.key
dest: /var/keys/nginx_signing.key
register: aptkey
- name: Add nginx apt key
command: "apt-key add /var/keys/nginx_signing.key"
when: aptkey.changed
- name: Update apt cache
apt:
update_cache: yes
when: aptkey.changed
4. Use Asynchronous Tasks
Asynchronous tasks allow playbooks to continue executing without waiting for long-running tasks to complete:
- Async and Poll: Use the
async
andpoll
keywords to run tasks asynchronously. For example:
- name: Long-running task
command: /path/to/long/task
async: 3600
poll: 0
register: task_result
- name: Check async task result
async_status:
jid: "{{ task_result.ansible_job_id }}"
register: job_result
until: job_result.finished
retries: 30
delay: 10
5. Enable Fact Caching
Fact gathering can be time-consuming, especially for large inventories. Enable fact caching to store and reuse host facts:
- Cache Backend: Configure a cache backend (e.g., JSON, Redis) in your
ansible.cfg
[defaults]
fact_caching = jsonfile
fact_caching_connection = /path/to/cache
6. Minimize the Use of wait_for
and pause
Excessive use of wait_for
and pause
can slow down playbook execution:
- Conditional Checks: Replace static pauses with conditional checks. For instance, wait for a port to open instead of pausing for a fixed duration:
- name: Wait for service to start
wait_for:
port: 8080
state: started
7. Profile Playbook Performance
Profiling helps identify bottlenecks in your playbooks:
- Callback Plugins: Use callback plugins like
profile_tasks
to measure the time taken by each task. Enable it in youransible.cfg
:
[defaults]
callback_whitelist = profile_tasks
8. Use Mitogen for Ansible
Mitogen is a third-party plugin for Ansible that can drastically improve execution speed by optimizing the communication layer between Ansible and remote hosts:
- First, install Mitogen via pip:
pip install mitogen ansible
Configure Mitogen: Modify your ansible.cfg
to use Mitogen as the strategy plugin:
[defaults]
strategy_plugins = /path/to/mitogen-ansible/ansible_mitogen/plugins/strategy
strategy = mitogen_linear
Mitogen can significantly reduce the overhead associated with Ansible’s SSH-based communication by using persistent connections and improving task execution efficiency.
Remember, the key to Ansible performance optimization lies in continuous monitoring and iterative improvement. Regularly profile your playbooks, analyze the results, and refine your configurations to achieve the best performance for your infrastructure.