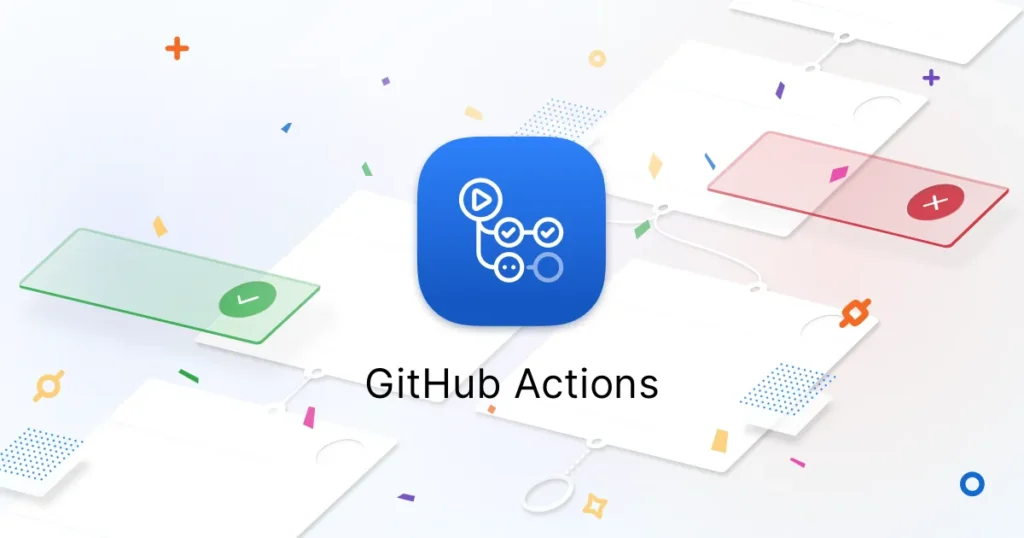
Pushing Docker images using GitHub Actions can streamline your deployment process. In this guide, we’ll show you how to push a Docker image to Docker Hub using GitHub Actions, step by step. You can also find a detailed example by visiting the GitHub repository here.
Before we start I assume that you have a basic understanding of Docker and Github.
What is GitHub actions:
GitHub Actions enables the user to create custom Software Development Life Cycle (SDLC) workflows in their GitHub repositories. It gives a privilege to the repository owner to write individual actions and then combine
them to create a custom workflow of their choice for their project. GitHub Actions also provides the facility to build end-to-end Continuous Integration (CI) and Continuous Deployment (CD) capabilities directly in the repository.
Let us take an example of how GitHub Action can be implemented in a simple repository.
Step 1
- Create a new repository
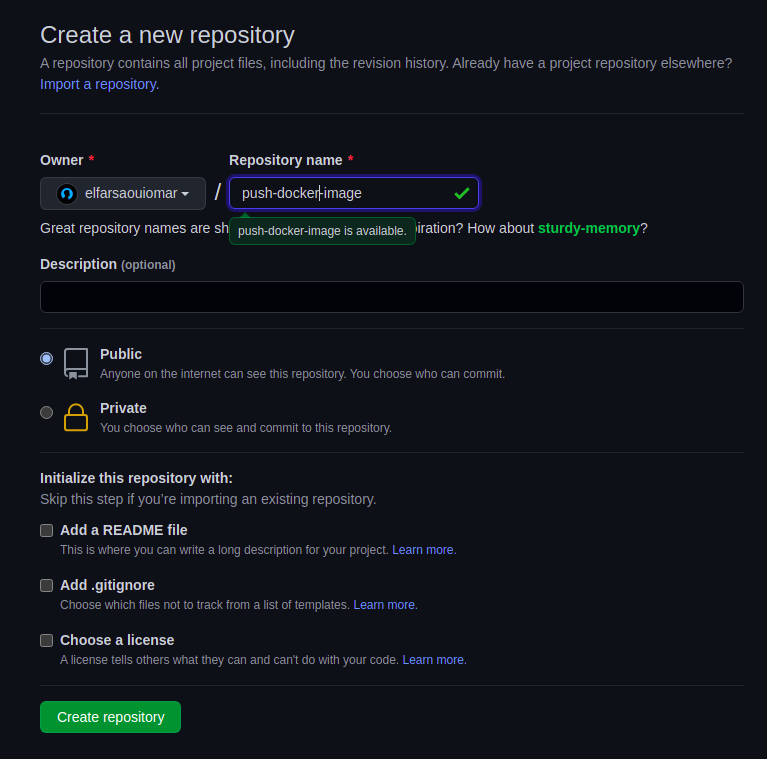
Create Dockerfile
nano Dockerfile
Paste this code in Dockerfile
FROM python:3.6ENV PYTHONDONTWRITEBYTECODE 1
ENV PYTHONUNBUFFERED 1
WORKDIR /code
ADD . /code
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
Then create app.py and paste this code below
from flask import Flaskapp = Flask(__name__)@app.route('/')
def home():
return 'Home Page'
if __name__ == "__main__":
app.run(host="0.0.0.0", port=8070, debug=False)
now let’s create a requirements.txt file to install the required python packages
nano requirements.txt
And also paste this code below.
flask=2.0.2
To make sure everything is working perfectly, run the following command.
$ docker build -t push-docker-image:latest .
then
docker run -d -p 8070:8070 push-docker-image:latest
Now check on the web browser
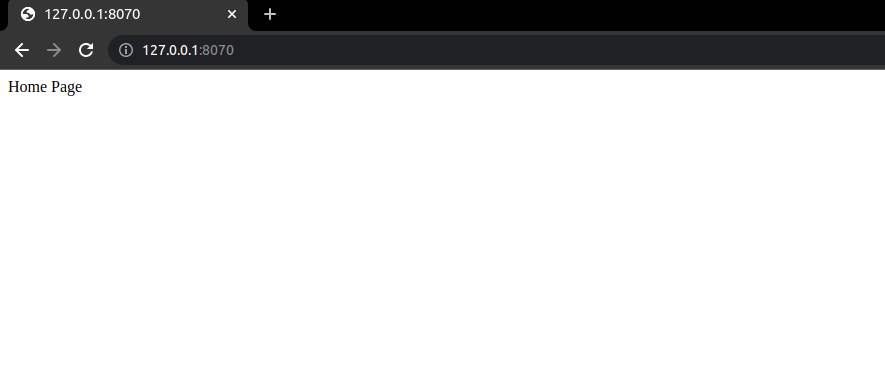
Step 2
Now I’ll create GitHub actions config file
- Create a .github/workflows folder.
mkdir -p .github/workflows
- and inside workflows folder create a .yml file, (eg push-docker-image.yaml)
touch .github/workflows/push-docker-image.yaml
Paste this code into your .yml file
name: Workflow Title
on:
push
env:
DOCKER_USER: ${{ secrets.DOCKER_USER }}
DOCKER_PASSWORD: ${{ secrets.DOCKER_PASSWORD }}
REPO_NAME: ${{ secrets.REPO_NAME }}
jobs:
push-image-to-docker-hub:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Docker Login
run: |
docker login -u $DOCKER_USER -p $DOCKER_PASSWORD
- name: Get Current Date
id: date
run: echo "::set-output name=date::$(date +'%Y-%m-%d--%M-%S')"
- name: Build Docker Image
run: docker build . --file Dockerfile --tag $DOCKER_USER/$REPO_NAME:${{ steps.date.outputs.date }}
- name: Push Docker Image
run: docker push $DOCKER_USER/$REPO_NAME:${{ steps.date.outputs.date }}
After pushing the code to GitHub, go to the Actions section, you will find the workflow you created, you will receive an error because those variables DOCKER_USER and DOCKER_PASSWORD and REPO_NAME are not set.
In order to fix that, go to settings –> secrets and create tree secrets (DOCKER_USER & DOCKER_PASSWORD, REPO_NAME), re-push an update and go back to the actions section you will now see a green circle witch means the workflow was completed successfully.
Now go to docker hub you will find an image with a tag similar to YYYY-M-D--m-s
.
congratulation we have successfully pushed an image to the docker hub.
Resources
- https://docs.github.com/en/actions
- https://www.beopenit.com/github-actions-le-nouveau-pipeline-ci-cd-de-github/
If you found this article on configuring security headers in Nginx valuable and want to keep up with the latest tips and best practices in web security, DevOps, and performance optimization, consider subscribing to our newsletter!